matplotlib图形绘制
类似于 gnuplot , matplotlib 也是开源的图形绘制软件。
需要使用到的工具如下:
- Jupyter:python 分布调试 和 Markdown 笔记记录一体化工具。
- Python3.10
- Python3 插件: matplotlib / pandas
- VS code 所需插件: pylance
其中, matplotlib 是绘图所用的开源工具库,而 pandas 是文档管理的 “瑞士军刀”。
环境安装
JUPYTER 安装
Jupyter可以支持在线和离线使用,在线即以网页形式支持使用,可以在服务器上进行部署。离线即在 Visual Studio Code 中以插件形式提供安装和使用。
在线安装
以 Ubuntu 系统为例,可以用 apt
进行安装,也可以用
pip
(或 pip3
)进行安装。
1 | sudo apt install jupyter |
在线版 Jupyter 还需要安装一个 ipyparallel
的类:
1 | pip3 install ipyparallel |
远程访问配置项
参考 《ubuntu 安装Juypter并设置远程访问》对 服务器端 Jupyter进行设置和访问测试。
相关防火墙设置 / 证书 / 域名 不再赘述。
自启动配置
另外,Linux 服务器端 Jupyter 用 jupyter notebook
命令启动,无法开机自启,需要参考 《Linux下Jupyter开机启动设置》
进行设置。
设置好后 在线版 Jupyter 编辑器的页面如下:
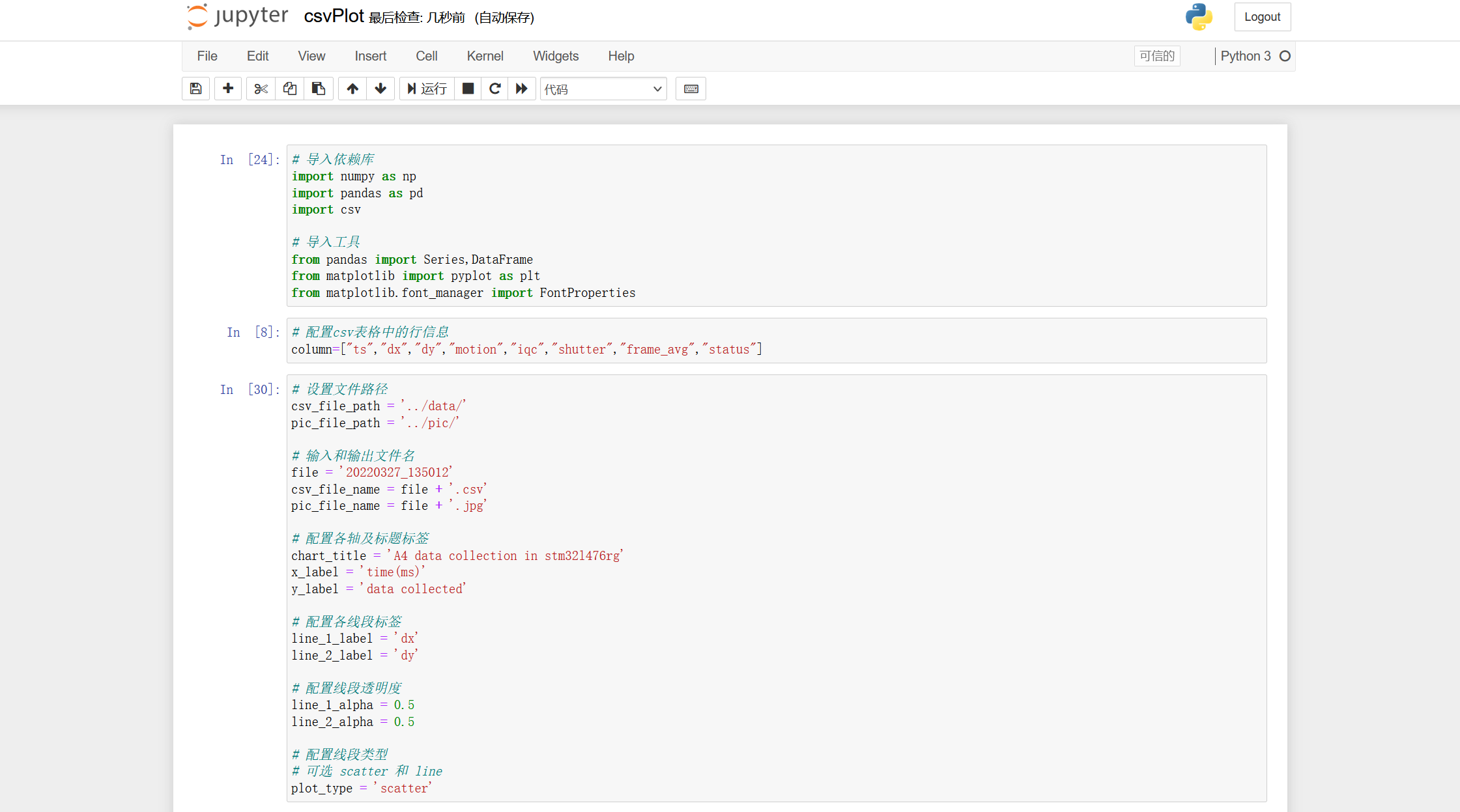
离线安装
在 VS code 插件中搜索并安装第一个 Jupyter
即可,插件系统会自动安装下面附带的 Jupyter Keymap
和
Jupyter Notebook Renderers
两个插件。
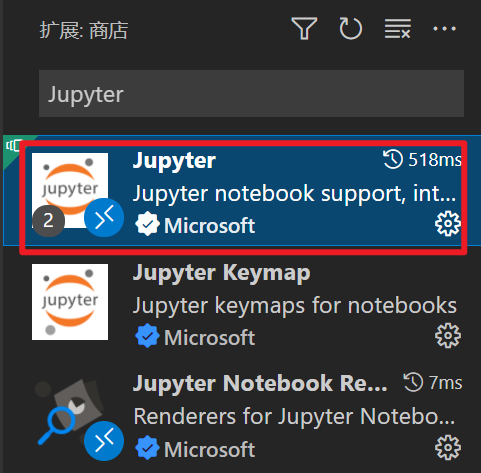
安装完之后,需要新建一个 .ipynb
格式的文件,即可自动打开
Jupyter
插件进行使用。
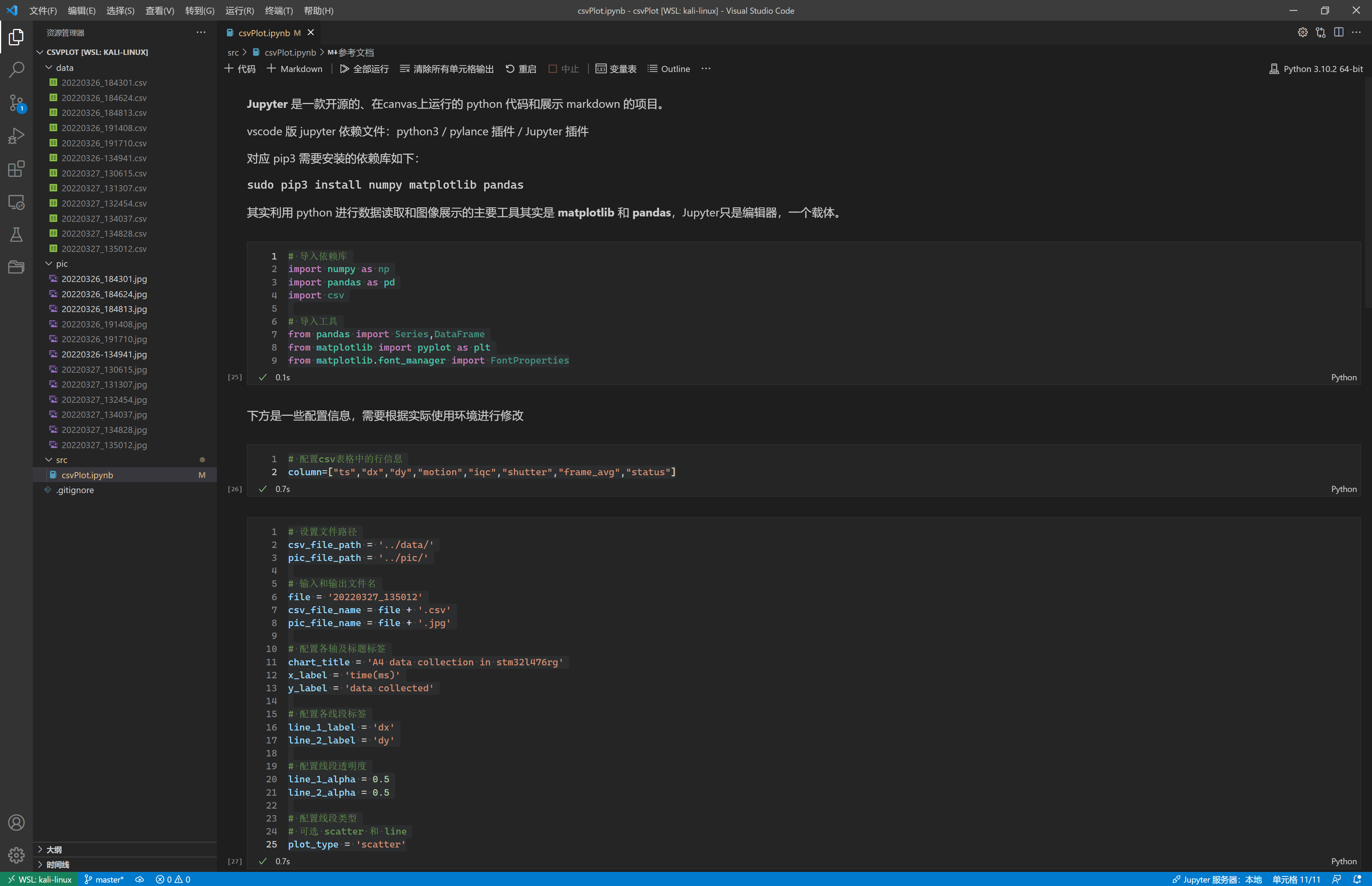
PIP 依赖库安装
在线版和离线版都需要使用 terminal 打开并输入命令进行安装下方的依赖库:
1 | pip3 install matplotlib pandas |
图形生成代码
下方利用 pandas 类对 .csv
文件中的相关列进行数据提取,然后利用 matplotlib 类对 数据进行绘图。
因功能比较简单,不再赘述。
具体需要注意的事项已在代码注释中写明。
1 | # 导入依赖库 |
使用方法
- 生成一个文件夹,下面包含三个并行子路径。
1 | ├── data |
网页版:
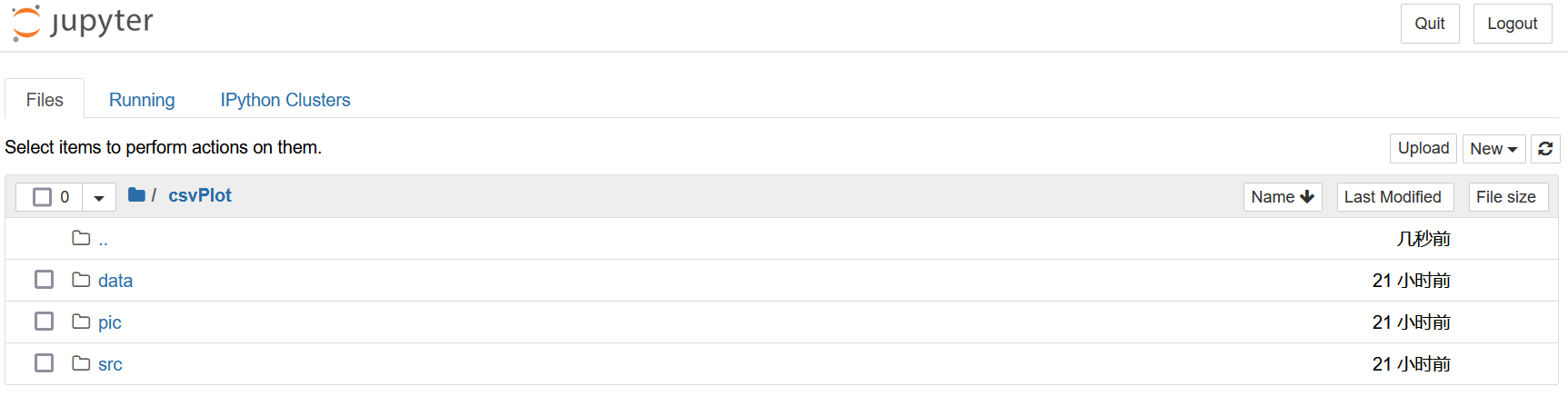
- 将代码放到
src/
中,在将代码第19行中的file = '20220327_135012'
文件名进行修改,确保其与data/
路径下的.csv
文件名成可以对上。 - 执行整段代码,即可在
pic/
文件夹下找到对应的图像文件。